ItemEvents.modifyTooltips
#Info
This event can be used from either client or server side.
To simply add tooltip to item you can use
event.add(filter, Text[])
method.You can register dynamic tooltip handler from server side but the handler itself has to be on client side.
Available methods in
ItemEvents.modifyTooltips
event:event.add(filter, Text[])
- shortcut method to just add text lines to itemevent.add(filter, TooltipRequirements, Text[])
- same method but 2nd argument adds requirements. See belowevent.modify(filter, (tooltip: TooltipActionBuilder) => void)
- modify tooltip with no requirementsevent.modify(filter, TooltipRequirements, (tooltip: TooltipActionBuilder) => void)
- modify tooltip with requirementsevent.modifyAll((tooltip: TooltipActionBuilder) => void)
- modify all tooltips with no requirementsevent.modifyAll(TooltipRequirements, (tooltip: TooltipActionBuilder) => void)
- modify all tooltips with requirements
#Basic Example
ItemEvents.modifyTooltips(event => {
// Show this tooltip for all red blocks
event.add(/minecraft:red_/, Text.red('Red!'))
// Only show this text when shift is not pressed
event.add('minecraft:campfire', {shift: false}, Text.gray('Campfire :D'))
// Only show this text when shift is pressed
event.add('minecraft:campfire', {shift: true}, Text.gray('Campfire :('))
})
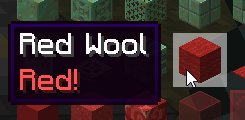
ItemEvents.modifyTooltips(event => {
event.modify('minecraft:pink_concrete_powder', tooltip => {
// Remove block title
tooltip.removeLine(0)
// Insert text at top of list
tooltip.insert(0, Text.of('funi block').color(0xFFA5F7))
})
})
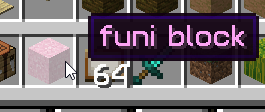
#Text actions
All available methods in
tooltip
of event.modify
:tooltip.dynamic(id)
tooltip.add(Text[])
- adds text lines at end of tooltiptooltip.insert(index, Text[])
- inserts text lines in middle of tooltip at specific linetooltip.removeLine(index)
- removes specific linetooltip.removeText(Text)
- removes line if any part of component's content matches inputtooltip.removeExactText(Text)
- removes line if it matches component exactlytooltip.clear()
- clears entire tooltip
Tooltip actions are executed in order, so if you call
tooltip.removeLine(0)
twice, it will remove first two lines#Tooltip Requirements
Available requirement properties:
shift: true/false
- if shift key is pressedctrl: true/false
- if ctrl key is pressedalt: true/false
- if alt key is pressedadvanced: true/false
- if advanced tooltips are enabled (F3+H)creative: true/false
- if player is in creative modestages: {stageName: true/false}
- if player has specific stage
You can combine multiple properties, e.g.
{shift: true, ctrl: false, advanced: true}